[ad_1]
On this article, we’ll develop a dapp (decentralized utility) with a token swap element. To finish this job, you’ll use your JavaScript expertise to construct a NodeJS backend and a ReactJS frontend. To cowl the DeFi blockchain improvement points, the next Web3 instruments will get you to the end line with out breaking a sweat:
The Moralis Web3 API to fetch on-chain information.The 1inch aggregator to implement the alternate options. Axios to bridge the information from the backend to the frontend.The wagmi library to implement Web3 authentication. MetaMask to connect with your DEX and check its functionalities.
Additionally, because of the Moralis Token API, you’ll be able to fetch real-time token costs utilizing the next traces of code:
const responseOne = await Moralis.EvmApi.token.getTokenPrice({
handle: question.addressOne
})
const responseTwo = await Moralis.EvmApi.token.getTokenPrice({
handle: question.addressTwo
})
So far as the blockchain improvement for DeFi options goes, these 1inch aggregator API endpoints will do the trick:
const allowance = await axios.get(`https://api.1inch.io/v5.0/1/approve/allowance?tokenAddress=${tokenOne.handle}&walletAddress=${handle}`)
const approve = await axios.get(`https://api.1inch.io/v5.0/1/approve/transaction?tokenAddress=${tokenOne.handle}`)
const tx = await axios.get(`https://api.1inch.io/v5.0/1/swap?fromTokenAddress=${tokenOne.handle}&toTokenAddress=${tokenTwo.handle}&quantity=${tokenOneAmount.padEnd(tokenOne.decimals+tokenOneAmount.size, ‘0’)}&fromAddress=${handle}&slippage=${slippage}`)
To implement the above code snippets, create your free Moralis account and observe our lead!
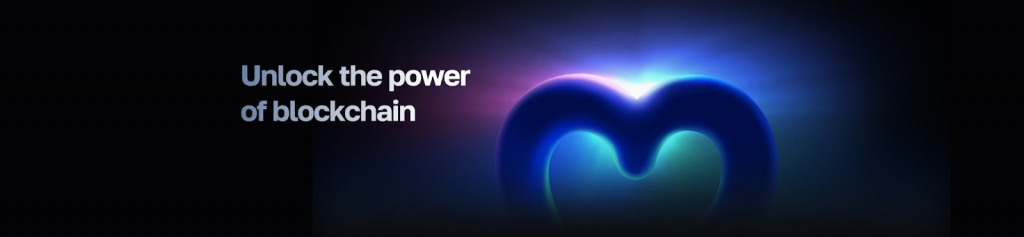
Overview
Within the first a part of right this moment’s article, you’ve gotten an opportunity to observe our lead and dive into DeFi blockchain improvement by creating your personal token swap dapp. Now, for those who determine to take action, you’ll be taught to arrange your venture, construct your DeFi dapp’s header, create a token swap web page, implement the backend DeFi performance, and guarantee your dapp interacts with the 1inch aggregator.
Under the tutorial, you’ll be able to be taught extra in regards to the theoretical points of blockchain improvement for DeFi initiatives.
DeFi Blockchain Improvement Tutorial: Construct a DEX
Decentralized exchanges (DEXs) are particular kinds of dapps that deliver DeFi to life. Whereas DEXs can have many options, all of them have a token swap. As such, that is the element of blockchain improvement for DeFi platforms we’ll deal with herein. Because the screenshot signifies, you don’t have to begin from zero. As an alternative, go to our GitHub repo web page and clone the “dexStarter” code:
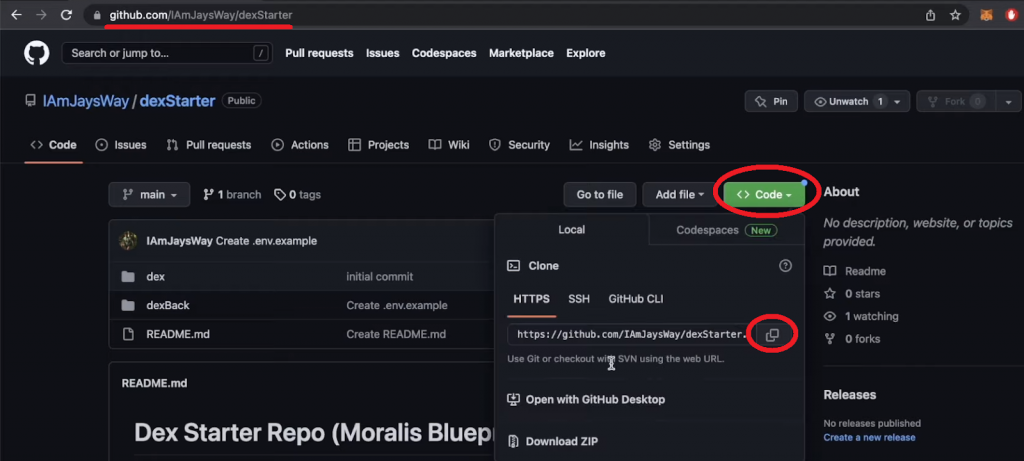
DeFi Undertaking Setup
Through the use of our starter venture, you don’t must cope with CSS styling; as a substitute, you’ll be able to commit your full consideration to the Web3 facet of DeFi blockchain improvement.
So, open a brand new venture in Visible Studio Code (VSC) and use your terminal to run the next command:
git clone https://github.com/IAmJaysWay/dexStarter
Then, navigate into the “dexStarter” listing. There, you’ll discover the “dex” and “dexBack” folders. The previous accommodates the template scripts to your dapp’s frontend, whereas the latter focuses on the backend portion of the venture. Primarily, you’re beginning with a easy ReactJS app for the frontend and a easy NodeJS app for the backend. Nevertheless, to be able to make these work, don’t overlook to put in the required dependencies. Begin along with your frontend (“cd” into “dex“) and run the next command:
npm set up
After putting in the dependencies, you can begin your React app with the command under:
npm run begin
Then you’ll be able to see the preliminary model of your DEX’s frontend by visiting “localhost:3000“:

Header of the Token Swap Dapp
Open the “App.js” script that awaits you within the “dex/src” listing. Subsequent, import the “Header” element on the high of the script:
import Header from “./parts/Header”;
Then, add the “Header” element to the “App” perform:
perform App() {
return (
<div className=”App”>
<Header />
</div>
)
}
Subsequent, entry the “Header.js” script from “dex/src/parts”. On the high of the file, import a brand and a series icon picture:
import Emblem from “../moralis-logo.svg”;
import Eth from “../eth.svg”;
Utilizing the next “Header” perform, you get to make sure that the script really shows the brand, the chain icon, web page choices, and the “Join” button:
perform Header(props) {
const {handle, isConnected, join} = props;
return (
<header>
<div className=”leftH”>
<img src={Emblem} alt=”brand” className=”brand” />
<div className=”headerItem”>Swap</div>
<div className=”headerItem”>Tokens</div>
</div>
<div className=”rightH”>
<div className=”headerItem”>
<img src={Eth} alt=”eth” className=”eth” />
Ethereum
</div>
<div className=”connectButton” onClick={join}>
{isConnected ? (handle.slice(0,4) +”…” +handle.slice(38)) : “Join”}
</div>
</div>
</header>
);
}
After tweaking “App.js” and “Header.js” as per the above instruction, it’s possible you’ll return to “localhost:3000” to view the progress:
The subsequent step is to assign correct routes to the “Swap” and “Tokens” choices. To take action, reopen the “App.js” script and import the next traces of code:
import Swap from “./parts/Swap”;
import Tokens from “./parts/Tokens”;
import { Routes, Route } from “react-router-dom”;
Then, tweak the “Header” div as follows:
<Header join={join} isConnected={isConnected} handle={handle} />
<div className=”mainWindow”>
<Routes>
<Route path=”/” component={<Swap isConnected={isConnected} handle={handle} />} />
<Route path=”/tokens” component={<Tokens />} />
</Routes>
</div>
Subsequent, return to the “Header.js” script to import “Hyperlink”:
import { Hyperlink } from “react-router-dom”;
Lastly, wrap the “Swap” and “Tokens” divs with the hyperlinks to the corresponding endpoint – the basis endpoint for the “Swap” web page and the “tokens” endpoint for the “Tokens” web page:
<Hyperlink to=”/” className=”hyperlink”>
<div className=”headerItem”>Swap</div>
</Hyperlink>
<Hyperlink to=”/tokens” className=”hyperlink”>
<div className=”headerItem”>Tokens</div>
</Hyperlink>
With the above tweaks in place, you’ll be able to once more discover the progress of your frontend:
The Token Swap Web page
Proceed this DeFi blockchain improvement tutorial by opening the “Swap.js” script. There, import the next Ant Design UI framework parts:
import React, { useState, useEffect } from “react”;
import { Enter, Popover, Radio, Modal, message } from “antd”;
import {
ArrowDownOutlined,
DownOutlined,
SettingOutlined,
} from “@ant-design/icons”;
Subsequent, tweak the “Swap” perform by including the “Slippage Tolerance” and “tradeBox” divs. Because of Ant Design, you’ll be able to simply implement a slippage settings function:
perform Swap() {
const [slippage, setSlippage] = useState(2.5);
perform handleSlippageChange(e) {
setSlippage(e.goal.worth);
}
const settings = (
<>
<div>Slippage Tolerance</div>
<div>
<Radio.Group worth={slippage} onChange={handleSlippageChange}>
<Radio.Button worth={0.5}>0.5%</Radio.Button>
<Radio.Button worth={2.5}>2.5%</Radio.Button>
<Radio.Button worth={5}>5.0%</Radio.Button>
</Radio.Group>
</div>
</>
);
return (
<div className=”tradeBox”>
<div className=”tradeBoxHeader”>
<h4>Swap</h4>
<Popover
content material={settings}
title=”Settings”
set off=”click on”
placement=”bottomRight”
>
<SettingOutlined className=”cog” />
</Popover>
</div>
</div>
</>
);
}
That is what the above additions to the “Swap.js” script appear like from the UI perspective:
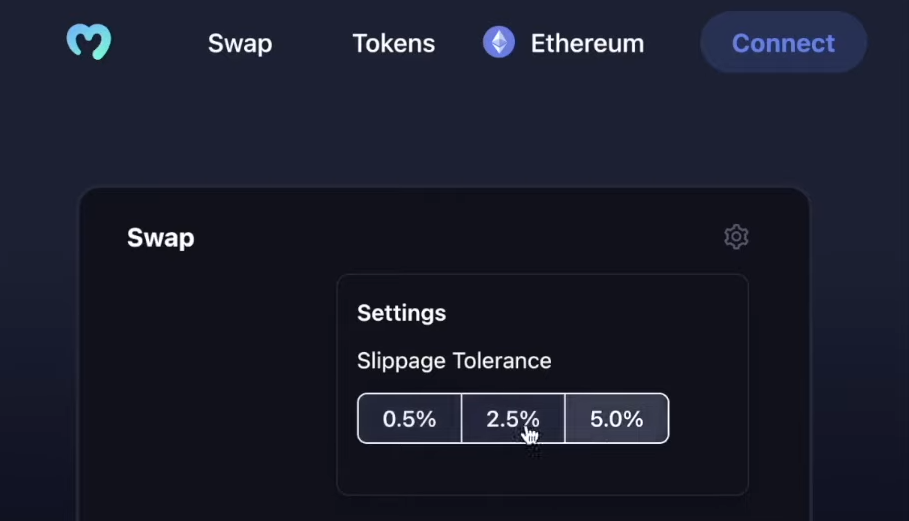
Including DEX Performance: Token Enter Fields
So far as the blockchain improvement for DeFi swaps goes, it’s good to create the enter fields the place customers can enter the variety of tokens they wish to alternate. So as to add these choices, tweak your “tradeBox” div by including the “inputs” div:
<div className=”inputs”>
<Enter placeholder=”0″ worth={tokenOneAmount} onChange={changeAmount} disabled={!costs} />
<Enter placeholder=”0″ worth={tokenTwoAmount} disabled={true} />
<div className=”switchButton” onClick={switchTokens}>
<ArrowDownOutlined className=”switchArrow” />
</div>
<div className=”assetOne” onClick={() => openModal(1)}>
<img src={tokenOne.img} alt=”assetOneLogo” className=”assetLogo” />
{tokenOne.ticker}
<DownOutlined />
</div>
<div className=”assetTwo” onClick={() => openModal(2)}>
<img src={tokenTwo.img} alt=”assetOneLogo” className=”assetLogo” />
{tokenTwo.ticker}
<DownOutlined />
</div>
</div>
To make the above traces of code work, you additionally want so as to add the next state variables under the “Slippage” state variable:
const [tokenOneAmount, setTokenOneAmount] = useState(null);
const [tokenTwoAmount, setTokenTwoAmount] = useState(null);
const [tokenOne, setTokenOne] = useState(tokenList[0]);
const [tokenTwo, setTokenTwo] = useState(tokenList[1]);
const [isOpen, setIsOpen] = useState(false);
const [changeToken, setChangeToken] = useState(1);
You additionally want correct capabilities to deal with the “from/to” switching of the tokens and altering the values within the entry fields. Thus, implement the capabilities under beneath the prevailing “handleSlippageChange” perform:
perform changeAmount(e) {
setTokenOneAmount(e.goal.worth);
if(e.goal.worth && costs){
setTokenTwoAmount((e.goal.worth * costs.ratio).toFixed(2))
}else{
setTokenTwoAmount(null);
}
}
perform switchTokens() {
setPrices(null);
setTokenOneAmount(null);
setTokenTwoAmount(null);
const one = tokenOne;
const two = tokenTwo;
setTokenOne(two);
setTokenTwo(one);
fetchPrices(two.handle, one.handle);
}
A DEX swap additionally wants to supply a correct choice of tokens. Therefore, you want an acceptable record of tokens that features their particulars, akin to token tickers, icons, names, addresses, and decimals. For that function, we created the “tokenList.json” file that awaits you contained in the “dex/src” folder:
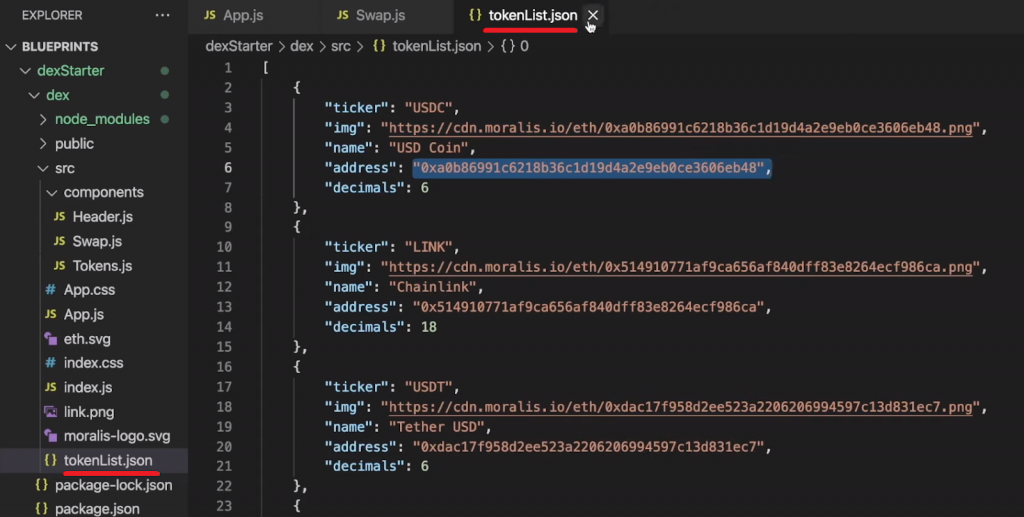
To import the above record, refocus on the “Swap.js” script and add the next line under the prevailing imports:
import tokenList from “../tokenList.json”;
Including DEX Performance: Token Choice Modals
Begin by including the next snippets of code to your “tradeBox” div (on the high of “return“):
return (
<>
{contextHolder}
<Modal
open={isOpen}
footer={null}
onCancel={() => setIsOpen(false)}
title=”Choose a token”
>
<div className=”modalContent”>
{tokenList?.map((e, i) => {
return (
<div
className=”tokenChoice”
key={i}
onClick={() => modifyToken(i)}
>
<img src={e.img} alt={e.ticker} className=”tokenLogo” />
<div className=”tokenChoiceNames”>
<div className=”tokenName”>{e.identify}</div>
<div className=”tokenTicker”>{e.ticker}</div>
</div>
</div>
);
})}
</div>
</Modal>
Subsequent, add the next “openModal” and “modifyToken” capabilities underneath the prevailing capabilities:
perform openModal(asset) {
setChangeToken(asset);
setIsOpen(true);
}
perform modifyToken(i){
setPrices(null);
setTokenOneAmount(null);
setTokenTwoAmount(null);
if (changeToken === 1) {
setTokenOne(tokenList[i]);
fetchPrices(tokenList[i].handle, tokenTwo.handle)
} else {
setTokenTwo(tokenList[i]);
fetchPrices(tokenOne.handle, tokenList[i].handle)
}
setIsOpen(false);
}
Lastly, your token swap field additionally wants the “Swap” button. For that, add the next line underneath the “inputs” div:
<div className=”swapButton” disabled= !isConnected onClick={fetchDexSwap}>Swap</div>
By implementing all the above tweaks, your frontend now seems like a correct DEX swap and awaits the backend functionalities:
Blockchain Improvement for DeFi Swap: Set Up a DEX Backend
For those who keep in mind the primary snippet of code from the intro, you understand it makes use of the Moralis “getTokenPrice” API endpoint. To make it work, nevertheless, it’s essential to get your Moralis Web3 API key. Luckily, it is a easy two-click course of when you log in to your Moralis account:
Contained in the “dexBack” folder, you’ll discover the “.env.instance” file. Open that file and substitute “GET YOURS FROM moralis.io” with the above-obtained API key. Additionally, rename “.env.instance” to “.env”. Then, open a brand new terminal to your backend and “cd” into the “dexBack” folder. As soon as in that folder, set up the backend dependencies by coming into the next command:
npm set up
To fetch token costs, it’s good to tweak the backend “index.js” script. So, open that script and implement the “Moralis.EvmApi.token.getTokenPrice” methodology for each tokens of the chosen buying and selling pair. Primarily, it’s good to replace the “app.get” perform as follows:
app.get(“/tokenPrice”, async (req, res) => {
const {question} = req;
const responseOne = await Moralis.EvmApi.token.getTokenPrice({
handle: question.addressOne
})
const responseTwo = await Moralis.EvmApi.token.getTokenPrice({
handle: question.addressTwo
})
const usdPrices = {
tokenOne: responseOne.uncooked.usdPrice,
tokenTwo: responseTwo.uncooked.usdPrice,
ratio: responseOne.uncooked.usdPrice/responseTwo.uncooked.usdPrice
}
return res.standing(200).json(usdPrices);
});
Observe: The ultimate “index.js” backend file is offered on GitHub in “dexBack” of the “dexFinal” repo:
Get Token Costs from the Backend to the Frontend
On this part of right this moment’s “DeFi blockchain improvement” tutorial, you’ll be taught to get the token costs from the above-presented backend to the previously-constructed “Swap” web page. So, return to the “Swap.js” script and import “axios” (under the prevailing imports):
import axios from “axios”;
Then, go to the a part of “swap.js” the place different state variables are situated and add the next:
const [prices, setPrices] = useState(null);
Subsequent, add the “fetchPrices” async perform under the “modifyToken” perform:
async perform fetchPrices(one, two){
const res = await axios.get(`http://localhost:3001/tokenPrice`, {
params: {addressOne: one, addressTwo: two}
})
setPrices(res.information)
}
You should additionally add the next “useEffect” under the above perform:
useEffect(()=>{
fetchPrices(tokenList[0].handle, tokenList[1].handle)
}, [])
With the above perform and “useEffect” in place, your “Swap” field UI can have the capability to make use of token costs and their ratios to robotically populate the quantity of the opposite token:
Implement Web3 Authentication
Now it’s time so as to add some performance to the “Join” button in your header. Because of the wagmi library, including Web3 login performance is fairly easy. Begin by opening your frontend “index.js” file from the “dex/src” folder. As soon as contained in the script, import the next wagmi parts and a public supplier underneath the prevailing imports:
import { configureChains, mainnet, WagmiConfig, createClient } from “wagmi”;
import { publicProvider } from “wagmi/suppliers/public”;
Subsequent, configure the chains and create a shopper by including this code snippet under the above imports:
const { supplier, webSocketProvider } = configureChains(
[mainnet],
[publicProvider()]
);
const shopper = createClient({
autoConnect: true,
supplier,
webSocketProvider,
});
You additionally must wrap your app with “WagmiConfig”:
<React.StrictMode>
<WagmiConfig shopper={shopper}>
<BrowserRouter>
<App />
</BrowserRouter>
</WagmiConfig>
</React.StrictMode>
Transferring ahead, reopen “App.js” and import the MetaMask connector and wagmi parts:
import { useConnect, useAccount } from “wagmi”;
import { MetaMaskConnector } from “wagmi/connectors/metaMask”;
Then, deal with the “App” perform and add the next traces of code above “return“:
const { handle, isConnected } = useAccount();
const { join } = useConnect({
connector: new MetaMaskConnector(),
});
Observe: For a extra detailed code walkthrough concerning the “Join” button performance, discuss with the video on the high of the article (57:25).
With the up to date frontend “index.js” and “App.js” scripts, the “Join” button triggers your MetaMask extension:
Implement the 1inch Aggregator
DeFi instruments just like the 1inch aggregator are extraordinarily highly effective as they permit you to make the most of decentralized options. With out these instruments, you’d must create and deploy your personal good contracts to realize the identical outcomes. With that mentioned, on this closing step of this DeFi blockchain improvement feat, it’s essential to implement the 1inch aggregator.
To make 1inch be just right for you, it’s good to add the 1inch API endpoints from the introduction and a few helping traces of code to the “Swap.js” script. The next 5 steps will take you to the end line:
Under the prevailing imports, import wagmi hooks:import { useSendTransaction, useWaitForTransaction } from “wagmi”; Add “props” to the “Swap” perform:perform Swap(props) {
const { handle, isConnected } = props; You additionally want extra state variables to retailer transaction particulars and look ahead to transactions to undergo:const [txDetails, setTxDetails] = useState({
to:null,
information: null,
worth: null,
});
const {information, sendTransaction} = useSendTransaction({
request: {
from: handle,
to: String(txDetails.to),
information: String(txDetails.information),
worth: String(txDetails.worth),
}
})
const { isLoading, isSuccess } = useWaitForTransaction({
hash: information?.hash,
}) Add the “fetchDexSwap” async perform with all of the required 1inch API endpoints underneath the “fetchPrices” perform:async perform fetchDexSwap(){
const allowance = await axios.get(`https://api.1inch.io/v5.0/1/approve/allowance?tokenAddress=${tokenOne.handle}&walletAddress=${handle}`)
if(allowance.information.allowance === “0”){
const approve = await axios.get(`https://api.1inch.io/v5.0/1/approve/transaction?tokenAddress=${tokenOne.handle}`)
setTxDetails(approve.information);
console.log(“not authorized”)
return
}
const tx = await axios.get(`https://api.1inch.io/v5.0/1/swap?fromTokenAddress=${tokenOne.handle}&toTokenAddress=${tokenTwo.handle}&quantity=${tokenOneAmount.padEnd(tokenOne.decimals+tokenOneAmount.size, ‘0’)}&fromAddress=${handle}&slippage=${slippage}`)
let decimals = Quantity(`1E${tokenTwo.decimals}`)
setTokenTwoAmount((Quantity(tx.information.toTokenAmount)/decimals).toFixed(2));
setTxDetails(tx.information.tx);
}
Observe: In case you are all for studying the place we obtained the above 1inch API hyperlinks, use the video on the high of the article, beginning at 1:09:10.
Final however not least, to cowl transaction particulars and pending transactions, add the next “useEffect” capabilities under the prevailing “useEffect” traces:useEffect(()=>{
if(txDetails.to && isConnected){
sendTransaction();
}
}, [txDetails])
useEffect(()=>{
messageApi.destroy();
if(isLoading){
messageApi.open({
kind: ‘loading’,
content material: ‘Transaction is Pending…’,
length: 0,
})
}
},[isLoading])
useEffect(()=>{
messageApi.destroy();
if(isSuccess){
messageApi.open({
kind: ‘success’,
content material: ‘Transaction Profitable’,
length: 1.5,
})
}else if(txDetails.to){
messageApi.open({
kind: ‘error’,
content material: ‘Transaction Failed’,
length: 1.50,
})
}
},[isSuccess])
Observe: You could find all closing scripts on our “dexFinal” GitHub repo web page.
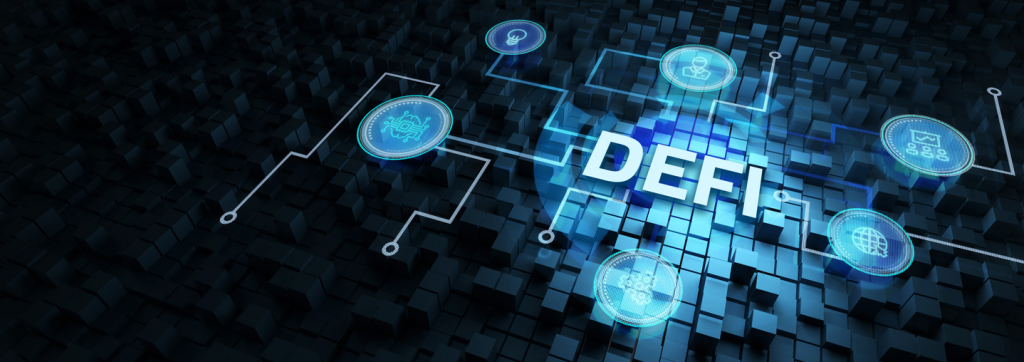
Blockchain Improvement for DeFi Tasks
Understanding the theoretical points of DeFi and blockchain improvement shouldn’t be important to construct a DeFi dapp. In spite of everything, for those who’ve adopted alongside in our tutorial above, you found that you can construct a DEX swap along with your JavaScript proficiency. Nevertheless, protecting the next fundamentals might be fairly helpful, and it’ll aid you sort out future DeFi blockchain improvement initiatives with extra confidence.
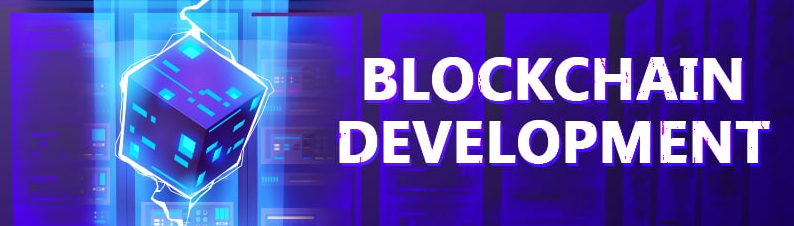
What’s Blockchain Improvement?
Blockchain improvement is the method of creating blockchain networks or different layers of the Web3 tech stack. So, the event of any Web3 instruments, platforms, good contracts, and all dapps matches this description. Primarily, if a venture incorporates blockchain tech indirectly, it falls underneath the scope of blockchain improvement.
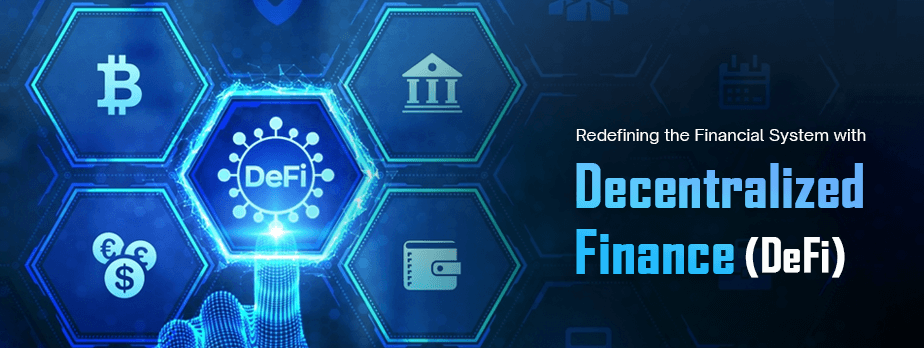
What’s Decentralized Finance (DeFi)?
DeFi, brief for decentralized finance, refers to a monetary system constructed on public blockchains with out a government and no intermediaries. These properties guarantee transparency and help peer-to-peer (P2P) buying and selling, borrowing, lending, and different monetary companies.
The final word objective of DeFi platforms is to permit customers to interact in all monetary actions that conventional markets provide however with none intermediaries. One of many key distinctions of DeFi companies is the truth that customers (friends) get to take part on each ends of the monetary companies. As such, DeFi is poised to remove the necessity for conventional monetary establishments.
DeFi and Blockchain Improvement
With blockchain tech at its core, DeFi is only one of many blockchain utilities/classes. Just like blockchain improvement on the whole, blockchain improvement for DeFi functions can goal totally different layers. There are numerous DeFi protocols that higher layers, akin to DeFi dapps, can make the most of. So, as a substitute of reinventing the wheel and writing your personal good contracts to deal with DeFi performance, you should use these protocols similar to we used the 1inch aggregator in right this moment’s tutorial. In fact, you too can construct from scratch.
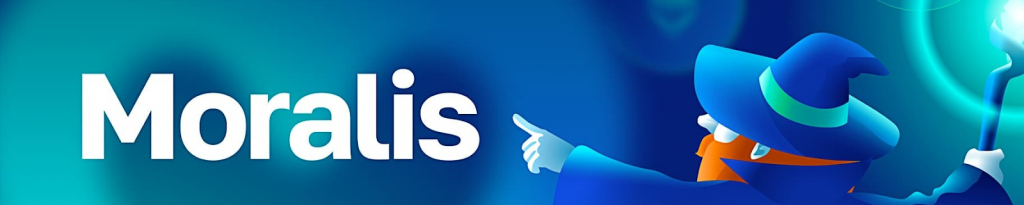
Methods to Get Began in DeFi Blockchain Improvement
There are a lot of methods to get began in DeFi blockchain improvement; nevertheless, the above tutorial is undoubtedly some of the frictionless paths. In spite of everything, it lets you use your legacy dev ability and canopy the blockchain-related backed facet utilizing Moralis, wagmi, and the 1inch aggregator.
Moralis lets you create all kinds of dapps utilizing a variety of Web3 APIs that aid you make the most of examined, dependable, and quick Web3 infrastructure. With the Moralis Web3 Knowledge API, you’ll be able to fetch all kinds of on-chain information, together with Ethereum logs and occasions. Plus, Moralis features a highly effective DeFi API, which comes within the type of the next two endpoints:
Get DEX token pair reserves:const response = await Moralis.EvmApi.defi.getPairReserves({
pairAddress,
chain,
}); Get DEX token pair addresses:const response = await Moralis.EvmApi.defi.getPairAddress({
token0Address,
token1Address,
chain,
});
As well as, you should use the Moralis Streams API to hearken to any good contract and pockets handle and use on-chain occasions as triggers to your DeFi dapps. Furthermore, because of Moralis’ cross-chain interoperability, you goal all of the main blockchains.
Observe: Study all it’s good to know in regards to the Moralis merchandise within the Moralis Web3 documentation.
With that in thoughts, Moralis, together with present main DeFi protocols, gives one of the simplest ways to begin creating unimaginable DeFi dapps the straightforward manner! In fact, when you determine to increase your dapps’ functionalities, you’ll additionally wish to be taught the fundamentals of good contract improvement. Nevertheless, even in the case of that, you don’t have to begin from scratch. As an alternative, you should use verified good contract templates provided by OpenZeppelin.
What Programming Language is Used for DeFi?
At this level, you understand that DeFi is an in depth department of blockchain functions. Since there are lots of methods to develop DeFi protocols and dapps, devs can use many programming languages. As an illustration, for those who take the trail of the least resistance and construct a DeFi dapp utilizing the above tutorial, JavaScript does the trick. Nevertheless, Moralis helps different main programming languages, so you can additionally use Python, Go, PHP, and so forth.
However, in the case of writing good contracts controlling on-chain DeFi actions, the language relies on the chain you deal with. So, for those who determine to deal with Ethereum and different EVM-compatible chains, Solidity is the best choice. Nevertheless, for those who’d prefer to create new DeFi functionalities on high of Solana or Aptos, the Rust and Transfer programming languages could be the go-to choices.
Though there isn’t a single programming language for DeFi, JavaScript (frontend and backend) and Solidity (good contracts) will provide you with essentially the most bang for the buck!
DeFi Blockchain Improvement – Methods to Develop DeFi Tasks – Abstract
In right this moment’s article, you had an opportunity to tackle our DeFi blockchain improvement tutorial and create your personal DEX swap dapp. Utilizing our template scripts, the facility of Moralis, wagmi, and the 1inch aggregator, you had been capable of get to the end line with out breaking a sweat. You additionally had a possibility to be taught what blockchain improvement and DeFi are and the way they match collectively. Nonetheless, you additionally realized methods to get began in DeFi blockchain improvement and what programming languages you’ll want on the way in which.
For those who want to discover different blockchain improvement matters, ensure to make use of the Moralis weblog. As an illustration, that is the place to be taught all it’s good to find out about a crypto faucet, Web3 ChatGPT, Alchemy NFT API, IPFS Ethereum, Solana blockchain app improvement, methods to create ERC20 token, and rather more. To spark some creativity, use our video tutorials that await you on the Moralis YouTube channel. Additionally, if you wish to turn out to be blockchain-certified, enroll in Moralis Academy.
[ad_2]
Source link